미리 알고 있어야 하는 것
Date.now()
- Date.now() 메소드는 UTC 기준으로 1970년 1월 1일 0시 0분 0초부터 현재까지 경과된 밀리초를 반환한다.
- 시간날짜를 얻는 함수 Date()와 while문 조건으로 3초가 지난걸 확인한다.
function wait(sec) {
let start = Date.now(), now = start;
while (now - start < sec * 1000) {
now = Date.now();
}
}
console.log(11)
wait(3);
console.log(22)
// 결과: 먼저 콘솔에 11이 찍힌다. 그 후, 3초가 지나고 22가 콘솔에 출력
Date.now() 가 3초를 세는 매커니즘
wait(3) -> let start = 현재시점, now = start;
while (now - start(현재시점) < 3 (초)) { now = 현재 시점에서 + 0.001 }
while (now - start(현재시점) < 3 (초)) { now = 현재 시점에서 + 0.0223 }
while (now - start(현재시점) < 3 (초)) { now = 현재 시점에서 + 0.1255601 }
while (now - start(현재시점) < 3 (초)) { now = 현재 시점에서 + 1.0356 }
.
. while 문은 촘촘히 반복된다 now 와 start의 차가 3이 막 넘을때까지!!!!!!!
.
while (now - start(현재시점) < 3 (초)) { now = 현재 시점에서 2.9999999 }
끝!!!
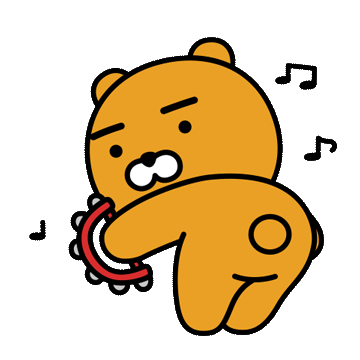
동기와 비동기 예시
동기 예시
function wait(ms) {
let start = Date.now(); // 1970.1.1. 0시 0분 0초부터 현재까지 경과된 밀리초를 나타내는 숫자
let now = start;
while(now - start < ms) {
now = Date.now();
}
}
function receive(person, coffee) {
console.log(person + '이(가) ' + coffee + '를 받았습니다.');
}
function orderCoffee(coffee) {
console.log(coffee + '가 접수되었습니다.');
wait(3000);
return coffee;
}
let customers = [{
name: 'min',
request: '돌체라떼',
}, {
name: 'jin',
request: '아메리카노'
},
{
name: 'lee',
request: '바닐라라떼'
}];
customers.forEach(function(customer){
let coffee = orderCoffee(customer.request);
receive(customer.name, coffee);
})
비동기 예시
function wait(callback, ms) {
setTimeout(callback, ms)
}
function receive(person, coffee) {
console.log(person + '이(가)' + coffee + '를 받았습니다.');
}
function orderCoffee(coffee, callback) {
console.log(coffee + '가 접수되었습니다.');
wait(function(){
callback(coffee);
}, 4000)
return coffee;
}
let customers = [{
name: 'min',
request: '돌체라떼',
}, {
name: 'jin',
request: '아메리카노'
},
{
name: 'lee',
request: '바닐라라떼'
}];
customers.forEach(function(customer){
orderCoffee(customer.request, function(coffee){
receive(customer.name, coffee);
})
})
DOM : 버튼에 비동기 이벤트 추가하기
function handleClick() {
console.log('버튼에 비동기 이벤트를 넣는 방법');
}
let button = document.createElement('button');
button.textContent = 'button';
document.body.append(button);
버튼에 비동기 이벤트를 연결하려면 함수 자체를 연결해야 한다. 3가지 방법이 있다.
// 첫 번째 방법
button.addEventListener('click', handleClick);
// 두 번째 방법
button.addEventListener('click', function() {
handleClick();
})
// 세 번째 방법
button.addEventListener('click', () => {
handleClick();
})
안 되는 경우
button.addEventListener('click', handleClick());
-> handleClick 함수를 실행한 것(undefined)을 연결하고 있기 때문에 정상적으로 작동하지 않는다.
728x90
'FE > JavaScript' 카테고리의 다른 글
[JS] fetch를 이용한 네트워크 요청 - 1/2 (개념) (0) | 2023.03.21 |
---|---|
[JS] Node.js 내장 모듈인 fs 모듈로 비동기 실습 - 1/2 (개념) (0) | 2023.03.21 |
[JS] 비동기 실습하기 (0) | 2023.03.21 |
[JS] fetch polyfill (0) | 2023.03.21 |
[JS] Axios (0) | 2023.03.21 |